The Node.js room of require()ment — Medium
Save article ToRead Archive Delete · Log in Log out
3 min read · View original · medium.com/@hikimberlyjane
The Node.js room of require()ment
Originally posted April 18, 2016
This post is intended to be helpful for beginners to Node.js and those at the intermediate level wanting to brush up on require().
One important fact to note is that require() is not part of your standard JavaScript. require() was built into Node.js to load modules, which is similar to include in C and import in Java. It’s also similar to adding in a piece of JavaScript code via a <script> tag. So what IS different? Unlike <script> , require() prevents leakage in the global scope. The file lives in its own scope, keeping in everything we defined in the file unless we explicitly expose specific functionality.
As an example, we have a module called multiply.js and an app.js where we usually keep our server code.
multiply.js (exporting a function):
‘use strict’;
function multiply(a,b){
return a * b;
}
module.exports = product;
app.js (requiring function):
‘use strict’;
var product = require(‘./multiply’)
console.log(product(2,2));
// result: 4
When you type node app.js on the command line, you should get 4. This, essentially, is require() in its most basic form.
npm is the Javascript developer’s Room of Requirement

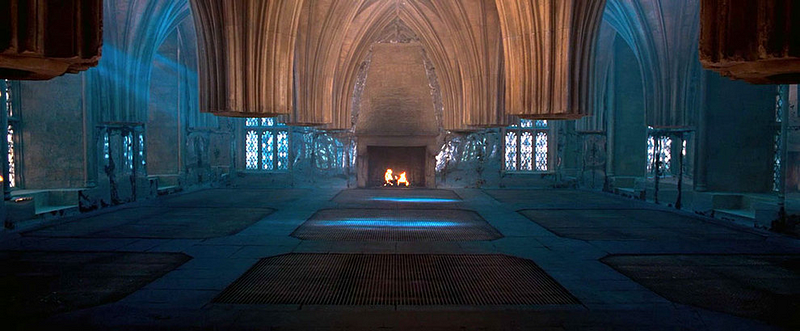
“Sometimes it is there, and sometimes it is not, but when it appears, it is always equipped for the seeker’s needs.” — Dobby explaining to Harry Potter the abilities of the Room of Requirement
According to the Node.js Documentation, require() was ‘designed to be general enough to support a number of reasonable directory structures.’ Require allows package managers like npm to build native packages from Node.js modules without modification.
npm is probably one of the most enjoyable things about developing in Node. I’m still amazed at how I can explore all the packages on npmjs.com and have access to code from thousands of developers, while being able to reassemble them in creative new ways. To dive into npm, you can look at some of the most starred packages at https://www.npmjs.com/browse/star
Start playing with npm
Note: npm comes automatically with node
Generate a package.json file.
npm init
Then, do an:
npm install colors —- save
You can specify the name of the package after ‘install’ in the command line. In this instance, we’d like to play with the colors package, which lets you get color and style in your node.js console.
Alternatively, you can also do -S
This will save the package as a dependency in the package.json file.
Next, you can require as a variable
var colors = require('colors');
Another important package to remember is request. It is essentially the AJAX of node, and it is one of the simplest ways to make calls. To experiment, you can check out some of the APIs out there, or go see the API directory at Programmable Web.
To start playing with an API, you can do:
npm i -S request
Then you would write:
var request = require(“request”);
To make a request to an API like the Star Wars API, you can start with a test.js file:
var request = require('request');
request(`http://swapi.co/api/people/1/`, (err, res, body) => { if(err) return console.log(err);
var people = JSON.parse(body)
console.log(people)
})
Then you can type in the terminal
node test.js
When you do this, what did you get?
If you got this guy, then you got it!

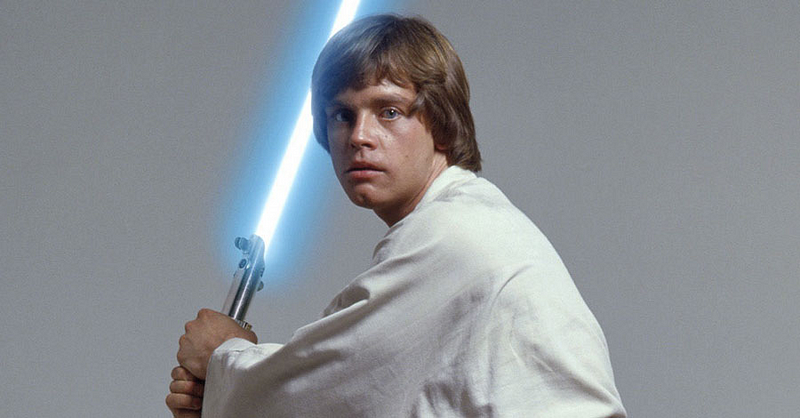
Require() in Node.js is essential for the Javascript developer’s toolbox. To me, npm is like the Room of Requirement in that it has everything you could ever ask for, and if you ever find yourself wondering, ‘Is there a package for that?’, there usually is.
Just like magic.